DISLIN Examples / C#
Demonstration of CURVE / C#
using System; public class App { public static void Main() { int n = 301; float [] xray = new float [n]; float [] y1ray = new float [n]; float [] y2ray = new float [n]; int i, ic; float x, step, fpi; fpi = 3.1415926f / 180; step = 360.0f / (n - 1); for (i = 0; i < n; i++) { xray[i] = i * step; x = xray[i] * fpi; y1ray[i] = (float) Math.Sin (x); y2ray[i] = (float) Math.Cos (x); } dislin.scrmod ("revers"); dislin.metafl ("xwin"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.axspos (450, 1800); dislin.axslen (2200, 1200); dislin.name ("X-axis", "X"); dislin.name ("Y-axis", "Y"); dislin.labdig (-1, "X"); dislin.ticks (10, "Y"); dislin.ticks (9, "X"); dislin.titlin ("Demonstration of CURVE", 1); dislin.titlin ("SIN(X), COS(X)", 3); ic = dislin.intrgb (0.95f, 0.95f, 0.95f); dislin.axsbgd (ic); dislin.graf (0.0f, 360.0f, 0.0f, 90.0f, -1.0f, 1.0f, -1.0f, 0.5f); dislin.setrgb (0.7f, 0.7f, 0.7f); dislin.grid (1, 1); dislin.color ("fore"); dislin.height (50); dislin.title (); dislin.color ("red"); dislin.curve (xray, y1ray, n); dislin.color ("green"); dislin.curve (xray, y2ray, n); dislin.disfin (); } }
Polar Plots / C#
using System; public class App { public static void Main() { int i, n = 300, m = 10, ic; float f = 3.1415927f / 180, step, a; float [] x1 = new float [n]; float [] y1 = new float [n]; float [] x2 = new float [m]; float [] y2 = new float [m]; step = 360.0f / (n - 1); for (i = 0; i < n; i++) { a = (i * step) * f; y1[i] = a; x1[i] = (float) Math.Sin (5 * a); } for (i = 0; i < m; i++) { x2[i] = i + 1; y2[i] = i + 1; } dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.setpag ("da4p"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.titlin ("Polar Plots", 2); dislin.ticks (3, "y"); dislin.axends ("NOENDS", "X"); dislin.labdig (-1, "Y"); dislin.axslen (1000, 1000); dislin.axsorg (1050, 900); ic = dislin.intrgb (0.95f, 0.95f, 0.95f); dislin.axsbgd (ic); dislin.grafp (1.0f,0.0f, 0.2f, 0.0f, 30.0f); dislin.color ("blue"); dislin.curve (x1, y1, n); dislin.color ("fore"); dislin.htitle (50); dislin.title (); dislin.endgrf (); dislin.labdig (-1, "X"); dislin.axsorg (1050, 2250); dislin.labtyp ("VERT", "Y"); dislin.grafp (10.0f, 0.0f, 2.0f, 0.0f, 30.0f); dislin.barwth (-5.0f); dislin.polcrv ("FBARS"); dislin.color ("blue"); dislin.curve (x2, y2, m); dislin.disfin (); } }
Symbols / C#
u sing System; using System.Text; public class App { public static void Main() { int nl, ny, i, nxp = 0; StringBuilder cstr = new StringBuilder (80); string ctit = "Symbols"; dislin.scrmod ("revers"); dislin.setpag ("da4p"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.height (60); nl = dislin.nlmess (ctit); dislin.messag (ctit, (2100 - nl) / 2, 200); dislin.height (50); dislin.hsymbl (120); ny = 150; for (i = 0; i < 24; i++) { if ((i % 4) == 0) { ny += 400; nxp = 550; } else { nxp += 350; } nl = dislin.intcha (i, cstr); nl = dislin.nlmess (cstr.ToString ()) / 2; dislin.messag (cstr.ToString (), nxp - nl, ny + 150); dislin.symbol (i, nxp, ny); } dislin.disfin (); } }
Interpolation Methods / C#
using System; using System.Text; public class App { public static void Main() { int nya = 2700, i, nx, ny, ic; float [] x = {0.0f, 1.0f, 3.0f, 4.5f, 6.0f, 8.0f, 9.0f, 11.0f, 12.0f, 12.5f, 13.0f, 15.0f, 16.0f, 17.0f, 19.0f, 20.0f}; float [] y = {2.0f, 4.0f, 4.5f, 3.0f, 1.0f, 7.0f, 2.0f, 3.0f, 5.0f, 2.0f, 2.5f, 2.0f, 4.0f, 6.0f, 5.5f, 4.0f}; string [] cpol = {"SPLINE", "STEM", "BARS", "STAIRS", "STEP", "LINEAR"}; string ctit = "Interpolation Methods"; dislin.setpag ("da4p"); dislin.metafl ("cons"); dislin.scrmod ("revers"); dislin.disini (); dislin.complx (); dislin.pagera (); dislin.incmrk (1); dislin.hsymbl (25); dislin.titlin (ctit, 2); dislin.axslen (1500, 350); dislin.setgrf ("line", "line", "line", "line"); ic = dislin.intrgb (1.0f, 1.0f, 0.0f); dislin.axsbgd (ic); for (i = 0; i < 6; i++) { dislin.axspos (350, nya - i * 350); dislin.polcrv (cpol[i]); dislin.marker (16); dislin.graf (0.0f, 20.0f, 0.0f, 5.0f, 0.0f, 10.0f, 0.0f, 5.0f); nx = dislin.nxposn (1.0f); ny = dislin.nyposn (8.0f); dislin.messag (cpol[i], nx, ny); dislin.color ("red"); dislin.curve (x, y, 16); dislin.color ("fore"); if (i == 5) { dislin.height (50); dislin.title (); } dislin.endgrf (); } dislin.disfin (); } }
Bar Graphs / C#
using System; using System.Text; public class App { public static void Main() { int nya = 2700, i; string ctit = "Bar Graphs (BARS)"; StringBuilder cbuf = new StringBuilder (25); float [] x = {1.0f, 2.0f, 3.0f, 4.0f, 5.0f, 6.0f, 7.0f, 8.0f, 9.0f}; float [] y = {0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f}; float [] y1 = {1.0f, 1.5f, 2.5f, 1.3f, 2.0f, 1.2f, 0.7f, 1.4f, 1.1f}; float [] y2 = {2.0f, 2.7f, 3.5f, 2.1f, 3.2f, 1.9f, 2.0f, 2.3f, 1.8f}; float [] y3 = {4.0f, 3.5f, 4.5f, 3.7f, 4.0f, 2.9f, 3.0f, 3.2f, 2.6f}; dislin.scrmod ("revers"); dislin.setpag ("da4p"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.ticks (1, "x"); dislin.intax ();; dislin.axslen (1600, 700); dislin.titlin (ctit, 3); dislin.legini (cbuf, 3, 8); dislin.leglin (cbuf, "FIRST", 1); dislin.leglin (cbuf, "SECOND", 2); dislin.leglin (cbuf, "THIRD", 3); dislin.legtit (" "); dislin.shdpat (5); for (i = 1; i <= 3; i++) { if (i > 1) dislin.labels ("none", "x"); dislin.axspos (300, nya - (i - 1) * 800); dislin.graf (0.0f, 10.0f, 0.0f, 1.0f, 0.0f, 5.0f, 0.0f, 1.0f); if (i == 1) { dislin.bargrp (3, 0.15f); dislin.color ("red"); dislin.bars (x, y, y1, 9); dislin.color ("green"); dislin.bars (x, y, y2, 9); dislin.color ("blue"); dislin.bars (x, y, y3, 9); dislin.color ("fore"); dislin.reset ("bargrp"); } else if (i == 2) { dislin.height (30); dislin.labels ("delta", "bars"); dislin.labpos ("center", "bars"); dislin.color ("red"); dislin.bars (x, y, y1, 9); dislin.color ("green"); dislin.bars (x, y1, y2, 9); dislin.color ("blue"); dislin.bars (x, y2, y3, 9); dislin.color ("fore"); dislin.reset ("height"); } else if (i == 3) { dislin.labels ("second", "bars"); dislin.labpos ("outside", "bars"); dislin.color ("red"); dislin.bars (x, y, y1, 9); dislin.color ("fore"); } if (i != 3) dislin.legend (cbuf, 7); if (i == 3) { dislin.height (50); dislin.title (); } dislin.endgrf (); } dislin.disfin (); } }
Pie Charts / C#
using System; using System.Text; public class App { public static void Main() { float [] xray = {1.0f, 2.5f, 2.0f, 2.7f, 1.8f}; StringBuilder cbuf = new StringBuilder (80); dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.setpag ("da4p"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.chnpie ("BOTH"); dislin.axslen (1600, 1000); dislin.titlin ("Pie Charts (PIEGRF)", 1); dislin.legini (cbuf, 5, 8); dislin.leglin (cbuf, "FIRST", 1); dislin.leglin (cbuf, "SECOND", 2); dislin.leglin (cbuf, "THIRD", 3); dislin.leglin (cbuf, "FOURTH", 4); dislin.leglin (cbuf, "FIFTH", 5); dislin.patcyc (1, 7); dislin.patcyc (2, 4); dislin.patcyc (3, 13); dislin.patcyc (4, 3); dislin.patcyc (5, 5); dislin.axspos (250, 2800); dislin.piegrf (cbuf.ToString (), 1, xray, 5); dislin.endgrf (); dislin.axspos (250, 1600); dislin.labels ("DATA", "PIE"); dislin.labpos ("EXTERNAL", "PIE"); dislin.piegrf (cbuf.ToString (), 1, xray, 5); dislin.height (50); dislin.title (); dislin.disfin (); } }
3-D Bar Graph / 3-D Pie Chart / C#
using System; using System.Text; public class App { public static void Main() { StringBuilder cbuf = new StringBuilder (80); float [] xray = {2.0f, 4.0f, 6.0f, 8.0f, 10.0f}; float [] y1ray = {0.0f, 0.0f, 0.0f, 0.0f, 0.0f}; float [] y2ray = {3.2f, 1.5f, 2.0f, 1.0f, 3.0f}; int [] ic1ray = {50, 150, 100, 200, 175}; int [] ic2ray = {50, 150, 100, 200, 175}; dislin.scrmod ("revers"); dislin.setpag ("da4p"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.titlin ("3-D Bar Graph / 3-D Pie Chart", 2); dislin.htitle (40); dislin.shdpat (16); dislin.axslen (1500, 1000); dislin.axspos (300, 1400); dislin.barwth (0.5f); dislin.bartyp ("3dvert"); dislin.labels ("second", "bars"); dislin.labpos ("outside", "bars"); dislin.labclr (255, "bars"); dislin.graf (0.0f, 12.0f, 0.0f, 2.0f, 0.0f, 5.0f, 0.0f, 1.0f); dislin.title (); dislin.color ("red"); dislin.bars (xray, y1ray, y2ray, 5); dislin.endgrf (); dislin.shdpat (16); dislin.labels ("data", "pie"); dislin.labclr (255, "pie"); dislin.chnpie ("none"); dislin.pieclr (ic1ray, ic2ray, 5); dislin.pietyp ("3d"); dislin.axspos (300, 2700); dislin.piegrf (cbuf.ToString (), 0, y2ray, 5); dislin.disfin (); } }
3-D Bars / BARS3D / C#
using System; using System.Text; public class App { public static void Main() { int n = 18, i; StringBuilder cbuf = new StringBuilder (80); float [] xwray = new float [n]; float [] ywray = new float [n]; float [] xray = {1.0f, 3.0f, 8.0f, 1.5f, 9.0f, 6.3f, 5.8f, 2.3f, 8.1f, 3.5f, 2.2f, 8.7f, 9.2f, 4.8f, 3.4f, 6.9f, 7.5f, 3.8f}; float [] yray = {5.0f, 8.0f, 3.5f, 2.0f, 7.0f, 1.0f, 4.3f, 7.2f, 6.0f, 8.5f, 4.1f, 5.0f, 7.3f, 2.8f, 1.6f, 8.9f, 9.5f, 3.2f}; float [] z1ray = {0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 0.0f}; float [] z2ray = {4.0f, 5.0f, 3.0f, 2.0f, 3.5f, 4.5f, 2.0f, 1.6f, 3.8f, 4.7f, 2.1f, 3.5f, 1.9f, 4.2f, 4.9f, 2.8f, 3.6f, 4.3f}; int [] icray = {30, 30, 30, 30, 30, 30, 100, 100, 100, 100, 100, 100, 170, 170, 170, 170, 170, 170}; for (i = 0; i < n; i++) { xwray[i] = (float) 0.5; ywray[i] = (float) 0.5; } dislin.scrmod ("revers"); dislin.setpag ("da4p"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.axspos (200, 2600); dislin.axslen (1800, 1800); dislin.name ("X-axis", "x"); dislin.name ("Y-axis", "y"); dislin.name ("Z-axis", "z"); dislin.titlin ("3-D Bars / BARS3D", 3); dislin.labl3d ("hori"); dislin.graf3d (0.0f, 10.0f, 0.0f, 2.0f, 0.0f, 10.0f, 0.0f, 2.0f, 0.0f, 5.0f, 0.0f, 1.0f); dislin.grid3d (1, 1, "bottom"); dislin.bars3d (xray, yray, z1ray, z2ray, xwray, ywray, icray, n); dislin.legini (cbuf, 3, 20); dislin.legtit (" "); dislin.legpos (1350, 1150); dislin.leglin (cbuf, "First", 1); dislin.leglin (cbuf, "Second", 2); dislin.leglin (cbuf, "Third", 3); dislin.legend (cbuf, 3); dislin.height (50); dislin.title (); dislin.disfin (); } }
Shading Patterns / C#
using System; using System.Text; public class App { public static void Main() { int [] ixp = new int [4]; int [] iyp = new int [4]; int [] ix = {0, 300, 300, 0}; int [] iy = {0, 0, 400, 400}; int nl, nx, nx0 = 335, ny0 = 350, ny, i, j, ii, k, iclr; StringBuilder cstr = new StringBuilder (80); string ctit = "Shading Patterns (AREAF)"; dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.setvlt ("small"); dislin.height (50); nl = dislin.nlmess (ctit); nx = (2970 - nl) / 2; dislin.messag (ctit, nx, 200); iclr = 0; for (i = 0; i < 3; i++) { ny = ny0 +i * 600; for (j = 0; j < 6; j++) { nx = nx0 + j * 400; ii = i * 6 + j; nl = dislin.intcha (ii, cstr); dislin.shdpat (ii); iclr = iclr % 16; iclr++; dislin.setclr (iclr); for (k = 0; k < 4; k++) { ixp[k] = ix[k] + nx; iyp[k] = iy[k] + ny; } dislin.areaf (ixp, iyp, 4); nl = dislin.nlmess (cstr.ToString ()); nx += (300 - nl) / 2; dislin.messag (cstr.ToString (), nx, ny + 460); } } dislin.disfin (); } }
3-D Colour Plot / C#
using System; public class App { public static void Main() { int n = 100; float [,] zmat = new float [n,n]; int i, j; float x, y, step, fpi; fpi = 3.1415926f / 180; step = 360.0f / (n - 1); for (i = 0; i < n; i++) { x = i * step; for (j = 0; j < n; j++) { y = j * step; zmat[i,j] = (float) (2 * Math.Sin (x * fpi) * Math.Sin (y * fpi)); } } dislin.scrmod ("revers"); dislin.metafl ("xwin"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.titlin ("3-D Colour Plot of the Function", 1); dislin.titlin ("F(X,Y) = 2 * SIN(X) * SIN(Y)", 3); dislin.name ("X-axis", "X"); dislin.name ("Y-axis", "Y"); dislin.name ("Z-axis", "Z"); dislin.axspos (300, 1850); dislin.ax3len (2200, 1400, 1400); dislin.intax (); dislin.autres (n, n); dislin.graf3 (0.0f, 360.0f, 0.0f, 90.0f, 0.0f, 360.0f, 0.0f, 90.0f, -2.0f, 2.0f, -2.0f, 1.0f); dislin.crvmat (zmat, n, n, 1, 1); dislin.height (50); dislin.title (); dislin.disfin (); } }
Surface Plot / C#
using System; public class App { public static void Main() { int n = 50, m = 50; float [,] zmat = new float [n,m]; int i, j; float x, y, step, fpi; fpi = 3.1415926f / 180; step = 360.0f / (n - 1); for (i = 0; i < n; i++) { x = i * step; for (j = 0; j < m; j++) { y = j * step; zmat[i,j] = (float) (2 * Math.Sin (x * fpi) * Math.Sin (y * fpi)); } } dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.setpag ("da4p"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.titlin ("Surface Plot of the Function", 2); dislin.titlin ("F(X,Y) = 2 * SIN(X) * SIN (Y)", 4); dislin.axspos (200, 2600); dislin.axslen (1800, 1800); dislin.name ("X-axis", "X"); dislin.name ("Y-axis", "Y"); dislin.name ("Z-axis", "Z"); dislin.view3d (-5.0f, -5.0f, 4.0f, "ABS"); dislin.graf3d (0.0f, 360.0f, 0.0f, 90.0f, 0.0f, 360.0f, 0.0f, 90.0f, -3.0f, 3.0f, -3.0f, 1.0f); dislin.height (50); dislin.title (); dislin.color ("green"); dislin.surmat (zmat, n, m, 1, 1); dislin.disfin (); } }
Shaded Surface Plot / C#
using System; public class App { public static void Main() { int n = 50, m = 50; float [,] zmat = new float [n,m]; float [] xray = new float [n]; float [] yray = new float [m]; int i, j; float x, y, step, fpi; fpi = 3.1415926f / 180; step = 360.0f / (n - 1); for (i = 0; i < n; i++) { x = i * step; xray[i] = x; for (j = 0; j < m; j++) { y = j * step; yray[j] = y; zmat[i,j] = (float) (2 * Math.Sin (x * fpi) * Math.Sin (y * fpi)); } } dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.setpag ("da4p"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.titlin ("Shaded Surface Plot", 2); dislin.titlin ("F(X,Y) = 2 * SIN(X) * SIN (Y)", 4); dislin.axspos (200, 2600); dislin.axslen (1800, 1800); dislin.name ("X-axis", "X"); dislin.name ("Y-axis", "Y"); dislin.name ("Z-axis", "Z"); dislin.view3d (-5.0f, -5.0f, 4.0f, "ABS"); dislin.graf3d (0.0f, 360.0f, 0.0f, 90.0f, 0.0f, 360.0f, 0.0f, 90.0f, -3.0f, 3.0f, -3.0f, 1.0f); dislin.height (50); dislin.title (); dislin.shdmod ("smooth", "surface"); dislin.surshd (xray, n, yray, m, zmat); dislin.disfin (); } }
Contour Plot / C#
using System; public class App { public static void Main() { int n = 50, m = 50; float [,] zmat = new float [n,m]; float [] xray = new float [n]; float [] yray = new float [m]; int i, j; float x, y, stepx, stepy, fpi, zlev; stepx = 360.0f / (n - 1); stepy = 360.0f / (m - 1); fpi = 3.1415926f / 180; for (i = 0; i < n; i++) { xray[i] = stepx * i; } for (j = 0; j < m; j++) { yray[j] = stepy * j; } for (i = 0; i < n; i++) { x = xray[i] * fpi; for (j = 0; j < m; j++) { y = yray[j] * fpi; zmat[i,j] = (float) (2 * Math.Sin (x) * Math.Sin (y)); } } dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.setpag ("da4p"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.titlin ("Contour Plot", 1); dislin.titlin ("F(X,Y) = 2 * SIN(X) * SIN(Y)", 3); dislin.intax (); dislin.axspos (450, 2650); dislin.name ("X-axis", "X"); dislin.name ("Y-axis", "Y"); dislin.graf (0.0f, 360.0f, 0.0f, 90.0f, 0.0f, 360.0f, 0.0f, 90.0f); dislin.height (50); dislin.title (); dislin.height (30); for (i = 0; i < 9; i++) { zlev = -2.0f + i * 0.5f; if (i == 4) dislin.labels ("NONE", "CONTUR"); else dislin.labels ("FLOAT", "CONTUR"); dislin.setclr ((i+1) * 28); dislin.contur (xray, n, yray, m, zmat, zlev); } dislin.disfin (); } }
Shaded Contour Plot / C#
using System; public class App { public static void Main() { int n = 50, m = 50, i, j; float [,] zmat = new float [n,m]; float [] xray = new float [n]; float [] yray = new float [m]; float [] zlev = new float [12]; string ctit1 = "Shaded Contour Plot"; string ctit2 = "F(X,Y) = (X[2$ - 1)[2$ + (Y[2$ - 1)[2$"; double x, y; double stepx = 1.6 / (n-1); double stepy = 1.6 / (m-1); for (i = 0; i < n; i++) { xray[i] = (float) (stepx * i); } for (j = 0; j < m; j++) { yray[j] = (float) (stepy * j); } for (j = 0; j < m; j++) { yray[j] = (float) (j * stepy); } for (i = 0; i < n; i++) { x = xray[i] * xray[i] - 1.0f; x *= x; for (j = 0; j < m; j++) { y = yray[j] * yray[j] - 1.0f; zmat[i,j] = (float) (x + y * y); } } dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.setpag ("da4p"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.mixalf (); dislin.titlin (ctit1, 1); dislin.titlin (ctit2, 3); dislin.name ("X-axis", "x"); dislin.name ("Y-axis", "y"); dislin.axspos (450, 2670); dislin.shdmod ("poly", "contur"); dislin.graf (0.0f, 1.6f, 0.0f, 0.2f, 0.0f, 1.6f, 0.0f, 0.2f); for (i = 0; i < 12; i++) { zlev[11-i] = 0.1f + i * 0.1f; } dislin.conshd (xray, n, yray, m, zmat, zlev, 12); dislin.height (50); dislin.title (); dislin.disfin (); } }
Shaded Surface / Contour Plot / C#
using System; public class App { public static void Main() { int n = 50, m = 50, i, j, nlev = 20; float [,] zmat = new float [n,m]; float [] xray = new float [n]; float [] yray = new float [m]; float [] zlev = new float [nlev]; double x, y, step; double fpi = 3.1415926/180.0; double stepx = 360.0 / (n-1); double stepy = 360.0 / (m-1); for (i = 0; i < n; i++) { x = i * stepx; xray[i] = (float) x; for (j = 0; j < m; j++) { y = j * stepy; yray[j] = (float) y; zmat[i,j] = (float) (2 * Math.Sin (x*fpi)* Math.Sin (y*fpi)); } } dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.setpag ("da4p"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.axspos (200, 2600); dislin.axslen (1800, 1800); dislin.name ("X-axis", "x"); dislin.name ("Y-axis", "y"); dislin.name ("Z-axis", "z"); dislin.titlin ("Shaded Surface / Contour Plot", 1); dislin.titlin ("F(X,Y) = 2*SIN(X)*SIN(Y)", 3); dislin.graf3d (0.0f, 360.0f, 0.0f, 90.0f, 0.0f, 360.0f, 0.0f, 90.0f, -2.0f, 2.0f, -2.0f, 1.0f); dislin.height (50); dislin.title (); dislin.grfini (-1.0f, -1.0f, -1.0f, 1.0f, -1.0f, -1.0f, 1.0f, 1.0f, -1.0f); dislin.nograf (); dislin.graf (0.0f, 360.0f, 0.0f, 90.0f, 0.0f, 360.0f, 0.0f, 90.0f); step = 4.0 / nlev; for (i = 0; i < nlev; i++) zlev[i] = (float) (-2.0 + i * step); dislin.conshd (xray, n, yray, n, zmat, zlev, nlev); dislin.box2d (); dislin.reset ("nograf"); dislin.grffin (); dislin.shdmod ("smooth", "surface"); dislin.surshd (xray, n, yray, m, zmat); dislin.disfin (); } }
Spheres and Tubes / C#
using System; public class App { public static void Main() { int i, j1, j2, iret; float [] x = {10.0f, 20.0f, 10.0f, 20.0f, 5.0f, 15.0f, 25.0f, 5.0f, 15.0f, 25.0f, 5.0f, 15.0f, 25.0f, 10.0f, 20.0f, 10.0f, 20.0f}; float [] y = {10.0f, 10.0f, 20.0f, 20.0f, 5.0f, 5.0f, 5.0f, 15.0f, 15.0f, 15.0f, 25.0f, 25.0f, 25.0f, 10.0f, 10.0f, 20.0f, 20.0f}; float [] z = {5.0f, 5.0f, 5.0f, 5.0f, 15.0f, 15.0f, 15.0f, 15.0f, 15.0f, 15.0f, 15.0f, 15.0f, 15.0f, 25.0f, 25.0f, 25.0f, 25.0f}; int [] idx = {1, 2, 1, 3, 3, 4, 2, 4, 5, 6, 6, 7, 8, 9, 9, 10, 11, 12, 12, 13, 5, 8, 8, 11, 6, 9, 9, 12, 7, 10, 10, 13, 14, 15, 16, 17, 14, 16, 15, 17, 1, 5, 2, 7, 3, 11, 4, 13, 5, 14, 7, 15, 11, 16, 13, 17}; dislin.setpag ("da4p"); dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.light ("on"); dislin.matop3 (0.02f, 0.02f, 0.02f, "specular"); dislin.clip3d ("none"); dislin.axspos (0, 2500); dislin.axslen (2100, 2100); dislin.htitle (50); dislin.titlin ("Spheres and Tubes", 4); dislin.name ("X-axis", "x"); dislin.name ("Y-axis", "y"); dislin.name ("Z-axis", "z"); dislin.labdig (-1, "xyz"); dislin.labl3d ("hori"); dislin.graf3d (0.0f, 30.0f, 0.0f, 5.0f, 0.0f, 30.0f, 0.0f, 5.0f, 0.0f, 30.0f, 0.0f, 5.0f); dislin.title (); dislin.shdmod ("smooth", "surface"); iret = dislin.zbfini(); dislin.matop3 (1.0f, 0.0f, 0.0f, "diffuse"); for (i = 0; i < 17; i++) dislin.sphe3d (x[i], y[i], z[i], 2.0f, 50, 25); dislin.matop3 (0.0f, 1.0f, 0.0f, "diffuse"); for (i = 0; i < 56; i += 2) { j1 = idx[i] - 1; j2 = idx[i+1] - 1; dislin.tube3d (x[j1], y[j1], z[j1], x[j2], y[j2], z[j2], 0.5f, 5, 5); } dislin.zbffin (); dislin.disfin (); } }
Some Solids / C#
using System; public class App { public static void Main() { int iret; dislin.setpag ("da4p"); dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.hwfont (); dislin.light ("on"); dislin.litop3 (1,0.5f, 0.5f, 0.5f, "ambient"); dislin.clip3d ("none"); dislin.axspos (0, 2500); dislin.axslen (2100, 2100); dislin.htitle (60); dislin.titlin ("Some Solids", 4); dislin.nograf (); dislin.graf3d (-5.0f, 5.0f, -5.0f, 2.0f, -5.0f, 5.0f, -5.0f, 2.0f, -5.0f, 5.0f, -5.0f, 2.0f); dislin.title (); dislin.shdmod ("smooth", "surface"); iret = dislin.zbfini(); dislin.matop3 (1.0f, 0.5f, 0.0f, "diffuse"); dislin.tube3d (-3.0f, -3.0f, 8.0f, 2.0f, 3.0f, 5.5f, 1.0f, 40, 20); dislin.rot3d (-60.0f, 0.0f, 0.0f); dislin.matop3 (1.0f, 0.0f, 1.0f, "diffuse"); dislin.setfce ("bottom"); dislin.matop3 (1.0f, 0.0f, 0.0f, "diffuse"); dislin.cone3d (-3.0f, -3.0f, 3.5f, 2.0f, 3.0f, 3.0f, 40, 20); dislin.setfce ("top"); dislin.rot3d (0.0f, 0.0f, 0.0f); dislin.matop3 (0.0f, 1.0f, 1.0f, "diffuse"); dislin.plat3d (4.0f, 4.0f, 3.0f, 3.0f, "icos"); dislin.rot3d (0.0f, 0.0f, 0.0f); dislin.matop3 (1.0f, 1.0f, 0.0f, "diffuse"); dislin.sphe3d (0.0f, 0.0f, 0.0f, 3.0f, 40, 20); dislin.rot3d (0.0f, 0.0f, -20.0f); dislin.matop3 (0.0f, 0.0f, 1.0f, "diffuse"); dislin.quad3d (-4.0f, -4.0f, -3.0f, 3.0f, 3.0f, 3.0f); dislin.rot3d (0.0f, 0.0f, 30.0f); dislin.matop3 (1.0f, 0.3f, 0.3f, "diffuse"); dislin.pyra3d (-2.0f, -5.0f, -10.0f, 3.0f, 5.0f, 5.0f, 4); dislin.rot3d (0.0f, 0.0f, 0.0f); dislin.matop3 (1.0f, 0.0f, 0.0f, "diffuse"); dislin.torus3d (7.0f, -3.0f, -2.0f, 1.5f, 3.5f, 1.5f, 0.0f, 360.0f, 40, 20); dislin.rot3d (0.0f, 90.0f, 0.0f); dislin.matop3 (0.0f, 1.0f, 0.0f, "diffuse"); dislin.torus3d (7.0f, -5.0f, -2.0f, 1.5f, 3.5f, 1.5f, 0.0f, 360.0f, 40, 20); dislin.zbffin (); dislin.disfin (); } }
Map Plot / C#
using System; public class App { public static void Main() { dislin.scrmod ("revers"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.axspos (400, 1850); dislin.axslen (2400, 1400); dislin.name ("Longitude", "X"); dislin.name ("Latitude", "Y"); dislin.titlin ("World Coastlines and Lakes", 3); dislin.labels ("MAP", "XY"); dislin.labdig (-1, "XY"); dislin.grafmp (-180.0f, 180.0f, -180.0f, 90.0f, -90.0f, 90.0f, -90.0f, 30.0f); dislin.gridmp (1,1); dislin.color ("green"); dislin.world (); dislin.color ("fore"); dislin.height (50); dislin.title (); dislin.disfin (); } }
TeX Instructions for Mathematical Formulas / C#
using System; public class App { public static void Main() { int nl; string cstr = "TeX Instructions for Mathematical Formulas"; dislin.scrmod ("revers"); dislin.setpag ("da4p"); dislin.metafl ("cons"); dislin.disini (); dislin.pagera (); dislin.complx (); dislin.height (40); nl = dislin.nlmess (cstr); dislin.messag (cstr, (2100 - nl)/2, 100); dislin.texmod ("on"); dislin.messag ("$\\frac{1}{x+y}$", 150, 400); dislin.messag ("$\\frac{a^2 - b^2}{a+b} = a - b$", 1200, 400); dislin.messag ("$r = \\sqrt{x^2 + y^2}", 150, 700); dislin.messag ("$\\cos \\phi = \\frac{x}{\\sqrt{x^2 + y^2}}$", 1200, 700); dislin.messag ("$\\Gamma(x) = \\int_0^\\infty e^{-t}t^{x-1}dt$", 150, 1000); dislin.messag ("$\\lim_{x \\to \\infty} (1 + \\frac{1}{x})^x = e$", 1200, 1000); dislin.messag ("$\\mu = \\sum_{i=1}^n x_i p_i$", 150, 1300); dislin.messag ("$\\mu = \\int_{-\\infty}^ \\infty x f(x) dx$", 1200, 1300); dislin.messag ("$\\overline{x} = \\frac{1}{n} \\sum_{i=1}^n x_i$", 150, 1600); dislin.messag ("$s^2 = \\frac{1}{n-1} \\sum_{i=1}^n (x_i - \\overline{x})^2$", 1200, 1600); dislin.messag ("$\\sqrt[n]{\\frac{x^n - y^n}{1 + u^{2n}}}$", 150, 1900); dislin.messag ("$\\sqrt[3]{-q + \\sqrt{q^2 + p^3}}$", 1200, 1900); dislin.messag ("$\\int \\frac{dx}{1+x^2} = \\arctan x + C$", 150, 2200); dislin.messag ("$\\int \\frac{dx}{\\sqrt{1+x^2}} = {\\rm arsinh} x + C$", 1200, 2200); dislin.messag ("$\\overline{P_1P_2} = \\sqrt{(x_2-x_1)^2 + (y_2-y_1)^2}$", 150,2500); dislin.messag ("$x = \\frac{x_1 + \\lambda x_2}{1 + \\lambda}$", 1200, 2500); dislin.disfin (); } }
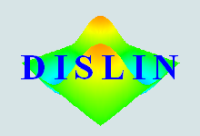
News
Upgrade 11.5.2
8. April 2024
Support for Python 3.11 and Windows
28. July 2023
Bug fix for the X11 distributions
22. July 2023
Upgrade 11.5.1
25. April 2023
Support for Linux 64-bit on IBM z series
30. October 2022
Support for MingW 64-bit UCRT runtime environment
28. September 2022
Release 11.5
15. March 2022
Release 11.4
15. March 2021
Support for Free Pascal 64-bit on Windows
22. July 2020
Upgrade 11.3.3
28. June 2020
DISLIN Book Version 11 is available
8. March 2017
8. April 2024
Support for Python 3.11 and Windows
28. July 2023
Bug fix for the X11 distributions
22. July 2023
Upgrade 11.5.1
25. April 2023
Support for Linux 64-bit on IBM z series
30. October 2022
Support for MingW 64-bit UCRT runtime environment
28. September 2022
Release 11.5
15. March 2022
Release 11.4
15. March 2021
Support for Free Pascal 64-bit on Windows
22. July 2020
Upgrade 11.3.3
28. June 2020
DISLIN Book Version 11 is available
8. March 2017